Scrollbar¶
There are 4 widgets who can have a scrollbar:
- Text()
- Treeview()
- Canvas()
- Listbox()
- Entry()
In order to add scrollbars to these widgets we define a class which adds them and use multiple inheritance.
In order for scrollbar and widget to interact, callback functions are assigned on both sides.
- The scrollbar calls the widget’s xview and yview methods.
- The widget has [x/y]scrollcommmand options to update the scrollbar
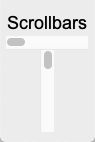
"""Display scrollbars."""
from tklib import *
class Demo(App):
def __init__(self):
super().__init__()
Label("Scrollbars", font="Arial 18")
ttk.Scrollbar(App.stack[-1], orient=tk.HORIZONTAL).grid()
ttk.Scrollbar(App.stack[-1], orient=tk.VERTICAL).grid()
Demo().run()
Listbox with scrollbars¶
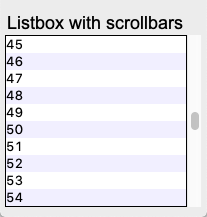
"""Listbox with a scrollbar."""
from tklib import *
class Demo(App):
def __init__(self):
super().__init__()
Label("Listbox with scrollbars", font="Arial 18")
lb = Listbox(list(range(100)))
sb = ttk.Scrollbar(App.stack[-1])
sb.grid(row=1, column=1, sticky='ns')
lb.config(yscrollcommand=sb.set)
sb.config(command=lb.yview)
Demo().run()
Text with scrollbars¶
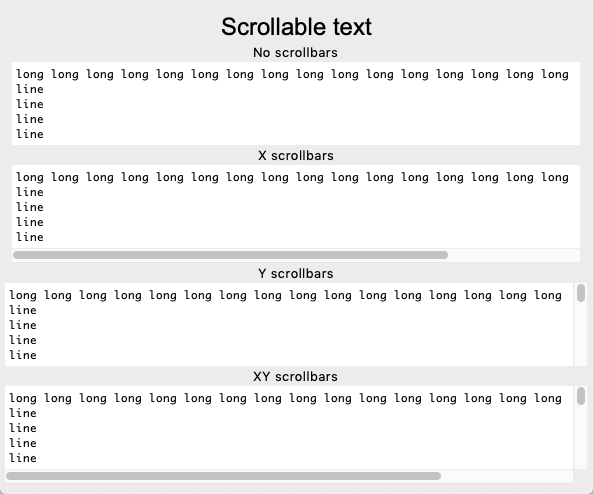
"""Text with a scrollbar."""
from tklib import *
class Demo(App):
def __init__(self):
super().__init__()
Label('Scrollable text', font='Arial 24')
text = 'long ' * 20
text += 'line\n' * 20
Label("No scrollbars")
Text(text, height=5, wrap='none')
Label("X scrollbars")
Text(text, height=5, scroll='x', wrap='none')
Label("Y scrollbars")
Text(text, height=5, scroll='y', wrap='none')
Label("XY scrollbars")
Text(text, height=5, scroll='xy', wrap='none')
Demo().run()
Canvas with scrollbars¶
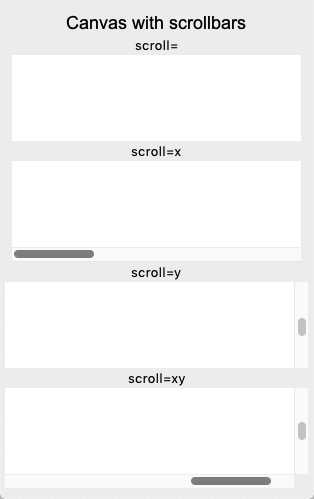
"""Canvas with a scrollbar."""
from tklib import *
class Canvas(tk.Canvas):
def __init__(self, scroll='', scrollregion=(0, 0, 1000, 1000), **kwargs):
self = Scrollable(tk.Canvas, scroll=scroll, scrollregion=scrollregion, **kwargs)
class Demo(App):
def __init__(self):
super().__init__()
text = 'hello ' * 100
Label("Canvas with scrollbars", font="Arial 18")
h = 80
Label('scroll=')
Canvas(height=h)
Label('scroll=x')
Canvas(height=h, scroll='x')
Label('scroll=y')
Canvas(height=h, scroll='y')
Label('scroll=xy')
Canvas(height=h, scroll='xy')
Demo().run()