Basic widgets¶
In this section we are going to look in more detail at these basic widgets:
- Button
- Label
- Entry
- Frame
Button¶
Buttons have text, a command and keyword arguements:
Button()
Button('Print 123', 'print(123)', padding=10)
Button('Hello', 'print("Hello " * 3)', default='active')
The first button has the default text Button and does nothing.
The second button has a padding of 10 pixels and prints
.!frame.!button2
The third button is active by default and prints::
Hello Hello Hello
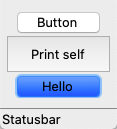
"""Create buttons with actions."""
from tklib import *
class Demo(App):
"""Create different buttons."""
def __init__(self):
super().__init__()
App.root.title('Button Demo')
Button()
Button('Print self', 'print(self)', padding=10)
Button('Hello', 'print("Hello " * 3)', default='active')
Demo().run()
Buttons which create buttons¶
Buttons can also be created or deleted dynamically:
- The first button creates another default button.
- The second button creates a self-destroy button.
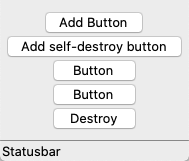
"""Buttons which create other buttons."""
from tklib import *
class Demo(App):
"""Create different buttons."""
def __init__(self):
super().__init__()
App.root.title('Button Demo')
Button('Add Button', 'Button()')
Button('Add self-destroy button', 'Button("Destroy", "self.destroy()")')
Demo().run()
Fonts¶
Labels can use different fonts and size.
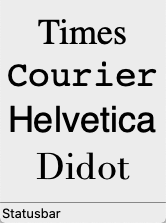
"""Show different fonts."""
from tklib import *
class Demo(App):
def __init__(self):
super().__init__()
fonts = ['Times', 'Courier', 'Helvetica', 'Didot']
for x in fonts:
Label(x, font=x+' 36')
Demo().run()
Display a label with an image with¶
Labels can be displayed with an additional image. The image position can be:
- center
- left/right
- top/bottom
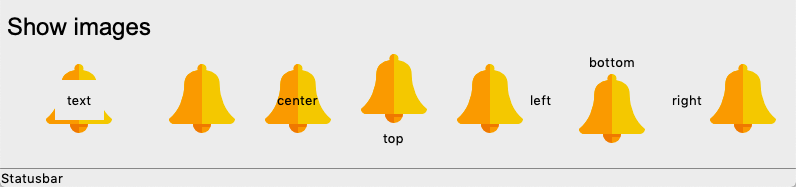
"""Display a label with an image."""
import os
from tklib import *
from PIL import Image, ImageTk
class Demo(App):
def __init__(self, **kwargs):
super().__init__(**kwargs)
Label('Show images', font='Arial 24')
lb = Label()
App.img0 = Image.open('icons/bell.png')
App.img = ImageTk.PhotoImage(App.img0)
lb['image'] = App.img
L = 'text;image;center;top;left;bottom;right'.split(';')
i = 0
for l in L:
lb = Label(l, compound=l, image=App.img, padding=10)
lb.grid(column=i, row=1)
i += 1
Demo().run()
Display a images as labels¶
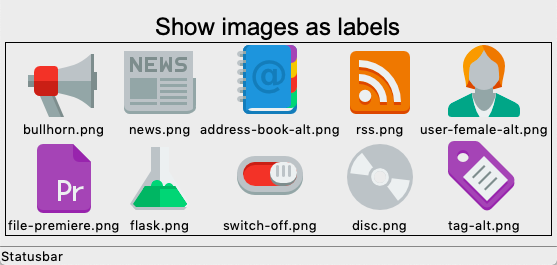
"""Display images as labels."""
from tklib import *
from PIL import Image, ImageTk
class Demo(App):
def __init__(self):
super().__init__()
Label('Show images as labels', font='Arial 24')
dir = os.listdir('icons')
n, m = 2, 5
self.images = []
Frame()
for i in range(n):
for j in range(m):
k = m*i + j
label = dir[k]
path = 'icons/' + label
img0 = Image.open(path)
img = ImageTk.PhotoImage(img0)
self.images.append(img)
lb = Label(image=img, text=label, compound='top')
lb.grid(row=i, column=j)
Demo().run()
Display a images as buttons¶
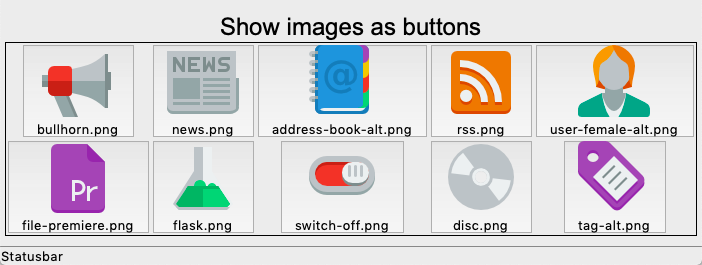
"""Display images as buttons."""
from tklib import *
from PIL import Image, ImageTk
class Demo(App):
def __init__(self, **kwargs):
super().__init__(**kwargs)
Label('Show images as buttons', font='Arial 24')
dir = os.listdir('icons')
n, m = 2, 5
self.images = []
Frame()
for i in range(n):
for j in range(m):
k = m*i + j
label = dir[k]
path = 'icons/' + label
img0 = Image.open(path)
img = ImageTk.PhotoImage(img0)
self.images.append(img)
lb = Button(image=img, text=label, compound='top')
lb.grid(row=i, column=j)
if __name__ == '__main__':
Demo().run()
Display a images in a listbox¶
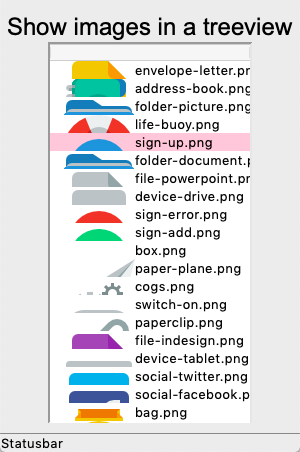
"""Display images as listbox."""
from tklib import *
from PIL import Image, ImageTk
class Demo(App):
def __init__(self, **kwargs):
super().__init__(**kwargs)
Label('Show images in a treeview', font='Arial 24')
dir = os.listdir('icons')
self.tree = Treeview(height=20)
self.images = []
for file in dir:
path = 'icons/' + file
img0 = Image.open(path)
img = ImageTk.PhotoImage(img0)
self.images.append(img)
self.tree.insert('', 'end', text=file, image=img)
Demo().run()
Embedded frames¶
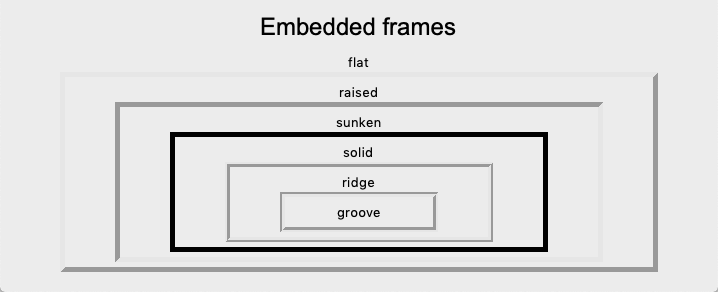
"""Embedded frames."""
from tklib import *
class Demo(App):
def __init__(self):
super().__init__()
Label('Embedded frames', font='Arial 24')
types = ['flat', 'raised', 'sunken', 'solid', 'ridge', 'groove']
for t in types:
Frame(relief=t, padding=(50, 5), borderwidth=5)
Label(t)
Demo().run()
Embedded frames¶
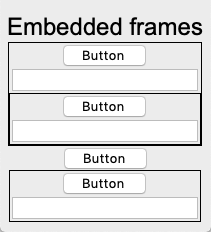
"""Speparate frames."""
from tklib import *
class Demo(App):
def __init__(self, **kwargs):
super().__init__(**kwargs)
Label('Embedded frames', font='Arial 24')
Frame()
Button()
Entry()
Frame()
Button()
Entry()
App.stack.pop()
App.stack.pop()
Button()
Frame()
Button()
Entry()
if __name__ == '__main__':
Demo().run()