Checkbutton¶
A checkbutton is like a regular button, with a label and a callback function, but it also holds and displays a binary state.
The Checkbutton
object has the following attributes:
- parent - the parent object
- text - the label to display
- command - the callback function
- variable - the variable holding the state value
- onvalue - the value when in the ON state
- offvalue - the value when in the OFF state
The standard checkbutton¶
Here is an example of 3 checkbuttons
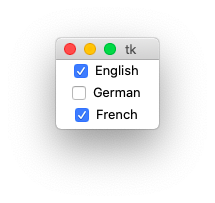
The callback function cb
writes this to the console:
--- languages ---
English 1
German 0
French fluent
We notice that the default offvalue is 0
and the default onvalue is 1
.
In our case:
- var0 toggles between
0
and1
- var1 toggles between
barely
and1
- var2 toggles between
0
andfluent
import tkinter as tk
root = tk.Tk()
var0 = tk.StringVar(value='1')
var1 = tk.StringVar(value='0')
var2 = tk.StringVar(value='0')
def cb():
print('--- languages ---')
print('English', var0.get())
print('German', var1.get())
print('French', var2.get())
tk.Checkbutton(root, text='English', variable=var0, command=cb).pack()
tk.Checkbutton(root, text='German', variable=var1, offvalue='barely', command=cb).pack()
tk.Checkbutton(root, text='French', variable=var2, onvalue='fluent', command=cb).pack()
root.mainloop()
Now let us rewrite this program by using lists.
import tkinter as tk
root = tk.Tk()
texts = ['English', 'German', 'French']
vars = [tk.StringVar(value='0'), tk.StringVar(value='0'), tk.StringVar(value='0')]
def cb():
print('--- languages ---')
for i, s in enumerate(texts):
print(s, vars[i].get())
tk.Checkbutton(root, text=texts[0], variable=vars[0], command=cb).pack()
tk.Checkbutton(root, text=texts[1], variable=vars[1], command=cb).pack()
tk.Checkbutton(root, text=texts[2], variable=vars[2], command=cb).pack()
root.mainloop()
A better Checkbutton class¶
It’s time now to define a new and better Checkbutton
class which can do everything in one line:
Checkbutton('English;German;French', 'print(self.selection)')
- the items are declared as a semicolon-separated list
- the command is a string to be evaluated in the Checkbutton environment
- the items are available in
self.items
- the selected items are available in
self.selection
- the selection states are available in
self.val
This is the result written to the console for three consecutive selections:
['French']
['German', 'French']
['English', 'German', 'French']
"""Create checkbuttons."""
from tklib import *
app = App()
Checkbutton('English;German;French', 'print(self.selection)')
app.run()
Now let’s see how this class is defined